Developer Guide
Use the Developer Guide to find the onboarding process steps, information about authentication methods, and a comprehensive list of the available ABC API endpoints.
Developer Guide
Authorization Methods
Sandbox
Production
Authorization Overview
ABC APIs use OAuth 2.0 for authorization of access to our endpoints. Depending on the type of integration (Individuals and Businesses or Third-Party Aggregator), the allowed scopes will vary based on the authorization grant used and are listed in the respective grant section below. The following grants are available:
- Authorization Code Flow
- Authorization Code Flow with Proof Key for Code Exchange (PKCE)
- Client Credentials for Third-Party Aggregators
- Client Credentials for Individuals and Businesses
The diagram below is a guide for choosing the appropriate authorization grant for your integration.
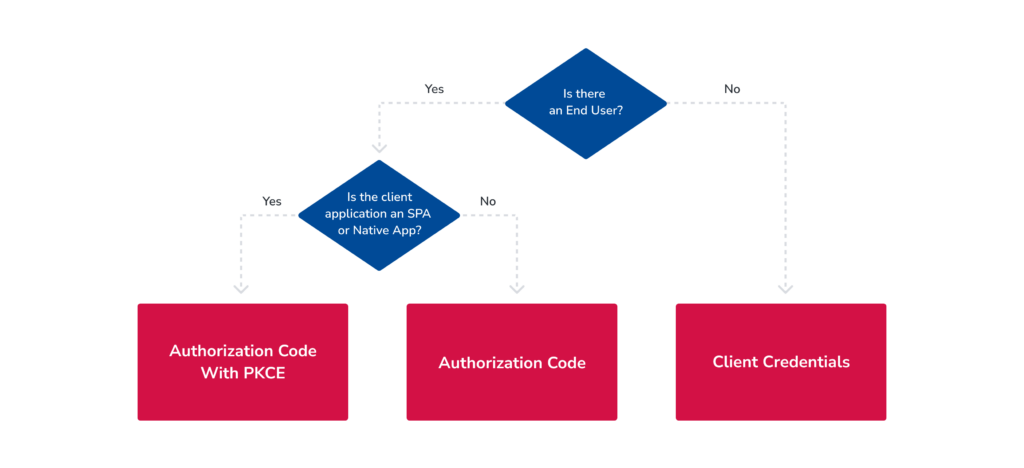
Authorization Code Flow
The authorization code flow is required when your application is taking actions on behalf of an ABC Supply customer.
This flow has the following steps:
- Your application requests an authorization code from the authorization server.
- The authorization server presents an authentication prompt to the user’s browser.
- The user signs in with their ABC Supply credentials and and provides consent.
- The browser receives an authorization code from the authorization server (Okta) after the user is authenticated. The authorization code is passed to your app.
- Your app sends this code and the client secret to the authorization server.
- The authorization server returns an access token, and optionally a refresh token.
- Your app can now use these tokens to call the connect partner API on behalf of the user.
- The ABC API validates the token before responding to the request.
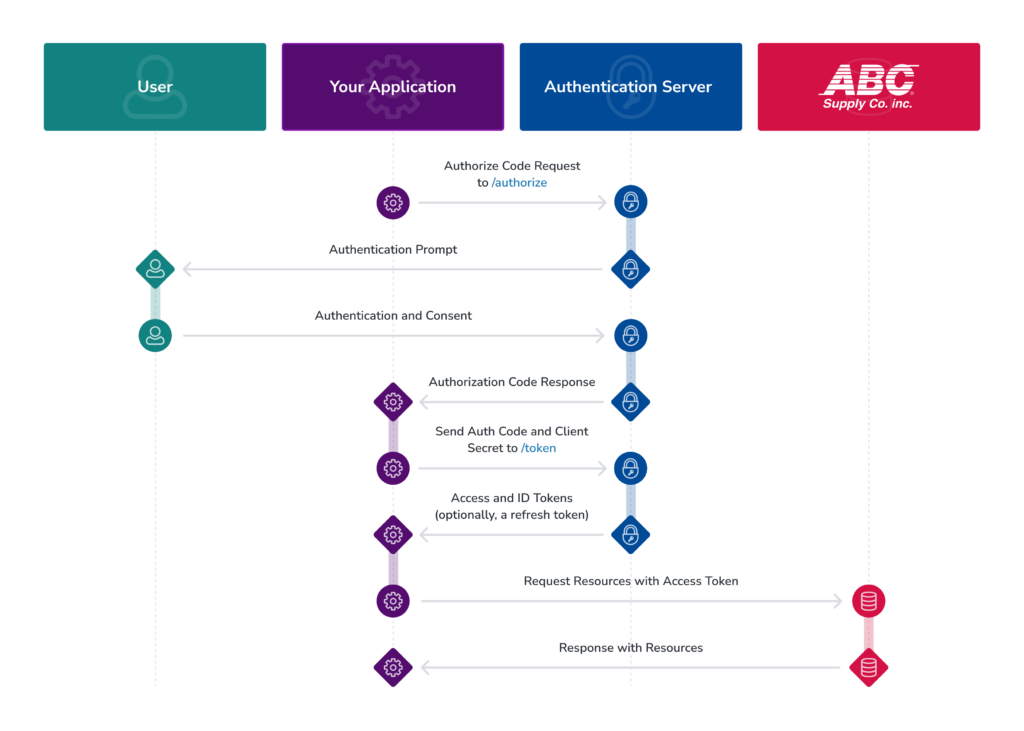
Example Authorization Request for Production
https://auth.partners.abcsupply.com/oauth2/ausvvp0xuwGKLenYy357/v1/authorize?
client_id={client_id}&
response_type=code&
redirect_uri={app_redirect_uri}&
state={random_string}&
scope=pricing.read%20order.read%20order.write%20product.read%20account.read%20location.read%20offline_access
Example Token Request for Production
curl -X POST \
'https://auth.partners.abcsupply.com/oauth2/ausvvp0xuwGKLenYy357/v1/token' \
-H 'Authorization: Basic {Base64(clientId:clientSecret)}' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'grant_type=authorization_code&redirect_uri={app_redirect_uri}&code={authorization_code_from_redirect}'
Example Refresh Token Request for Production
curl -X POST \
'https://auth.partners.abcsupply.com/oauth2/ausvvp0xuwGKLenYy357/v1/token' \
-H 'Authorization: Basic {Base64(clientId:clientSecret)}' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'grant_type=refresh_token&refresh_token={your_previously_obtained_refresh_token}&scope=pricing.read%20order.read%20order.write%20product.read%20account.read%20location.read%20offline_access'
Available Scopes
Scope Name
Description
location.read
Allows access to Location API
product.read
Allows access to Product API
account.read
Allows access to Account API
pricing.read
Allows access to Pricing API
order.read
Allows read-access to the Order API for orders placed through the integration
order.write
Allows write-access to Order API for placing orders
notification.read
Allows read-access for Notification API
notification.write
Allows write-access for Notification API
offline_access
Allows Refresh Token to be sent back by the token endpoint
Authorization Code Flow with PKCE
At a high level, the flow has the following steps:
- Your application generates a code verifier followed by a code challenge. See Create the proof key for code exchange.
- Your app requests an authorization code from the authorization server (Okta). The request contains the generated code challenge.
- The authorization server presents an authentication prompt to the user’s browser.
- The user signs in with their ABC Supply credentials and and provides consent.
- Okta redirects back to your application with an authorization code.
- Your application sends this code, along with the code verifier, to Okta. See Exchange the code for tokens.
- The authorization server evaluates the PKCE code.
- The authorization server returns an access token, and optionally a refresh token.
- Your app can now use these tokens to call the connect partner API on behalf of the user.
- The ABC API validates the token before responding to the request.
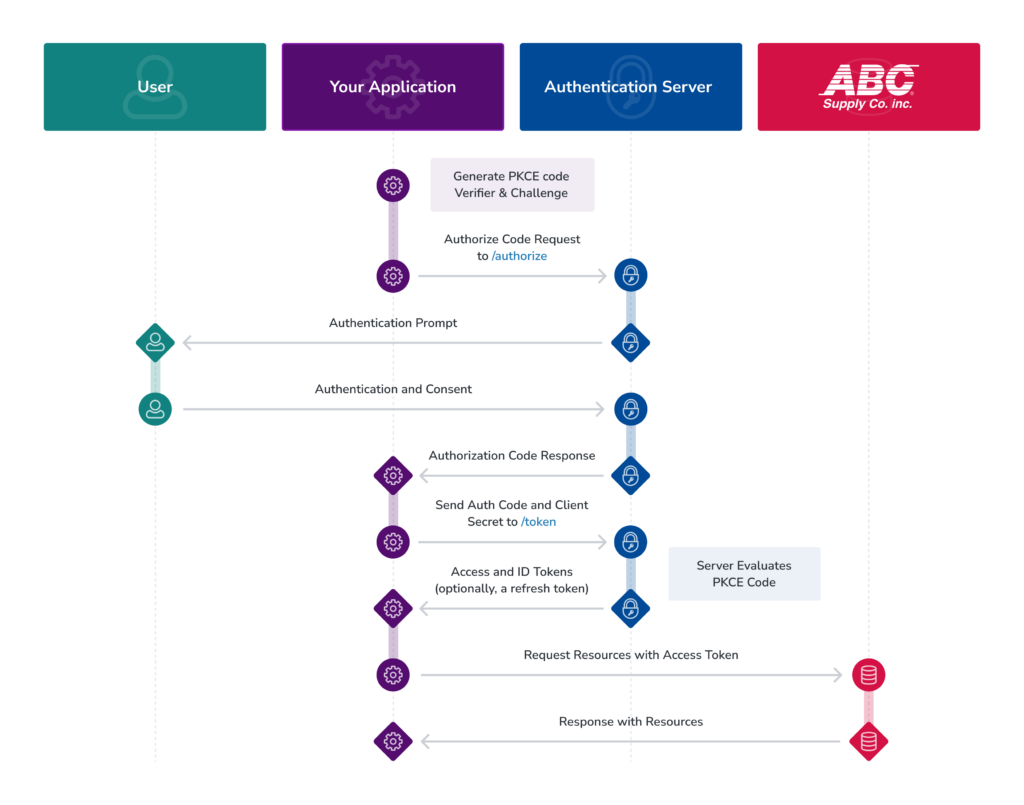
Example Authorization Request for Production
https://auth.partners.abcsupply.com/oauth2/ausvvp0xuwGKLenYy357/v1/authorize?
client_id={client_id}&
response_type=code&
redirect_uri={app_redirect_uri}&
state={random_string}&
code_challenge={base_64_encoded_sha_256_of_code_verifier}&
code_challenge_method=S256&
scope=pricing.read%20order.read%20order.write%20product.read%20account.read%20location.read%20offline_access
Example Token Request for Production
curl -X POST \
'https://auth.partners.abcsupply.com/oauth2/ausvvp0xuwGKLenYy357/v1/token' \
-H 'Authorization: Basic {Base64(clientId:clientSecret)}' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'grant_type=authorization_code&redirect_uri={app_redicrect_uri}&code={authorization_code_from_redirect}&code_verifier={random_string_generated_by_client}'
Example Refresh Token Request for Production
curl -X POST \
'https://auth.partners.abcsupply.com/oauth2/ausvvp0xuwGKLenYy357/v1/token' \
-H 'Authorization: Basic {Base64(clientId:clientSecret)}' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'grant_type=refresh_token&refresh_token={refresh_token_issued}&scope=pricing.read%20order.read%20order.write%20product.read%20account.read%20location.read%20offline_access'
Available Scopes
Scope Name
Description
location.read
Allows access to the Location API
product.read
Allows access to the Products API
account.read
Allows access to the Accounts API
pricing.read
Allows access to the Pricing API
order.read
Allows access to the Order API (GET Endpoint)
order.write
Allows write-access to Order API for placing orders
notification.read
Allows read-access for Notification API
notification.write
Allows write-access for Notification API
offline_access
Allows Refresh Token to be sent back by the token endpoint
Client Credentials for Third Party Aggregators
The Client Credentials flow is used when client applications request an access token on behalf of the application itself.
At a high level, the flow has the following steps:
- Your client application makes an authorization request to the authorization server using its client credentials.
- The authorization server responds with an access token if the request credentials are accurate.
- Your app uses the access token to make authorized requests to the resource server.
- The resource server validates the token before responding to the request.
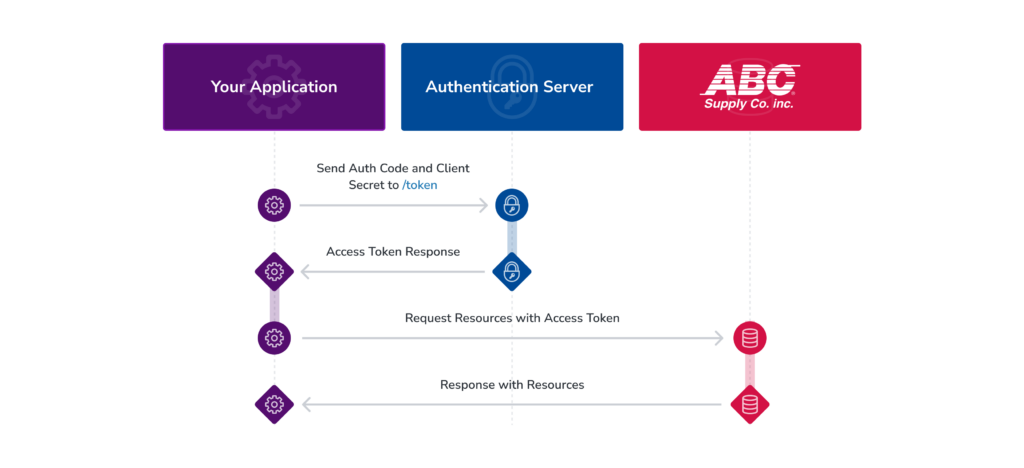
Example Token Request for Production
curl -X POST \
'https://auth.partners.abcsupply.com/oauth2/ausvvp0xuwGKLenYy357/v1/token' \
-H 'Authorization: Basic {Base64(clientId:clientSecret)}' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'grant_type=client_credentials&scope=location.read product.read notification.read notification.write'
Note: There is no authorization or refresh token call with the Client Credentials flow.
Available Scopes
Scope Name
Description
location.read
Allows access to the Location API
product.read
Allows access to the Products API
notification.read
Allows access to the Notification API (GET endpoint)
notification.write
Allows access to the Notification API (POST/Delete endpoints)
Client Credentials for Individuals and Businesses
The Client Credentials flow is used when client applications request an access token on behalf of the application itself.
At a high level, the flow has the following steps:
- Your client application makes an authorization request to the authorization server using its client credentials.
- The authorization server responds with an access token if the request credentials are accurate.
- Your app uses the access token to make authorized requests to the resource server.
- The resource server validates the token before responding to the request.
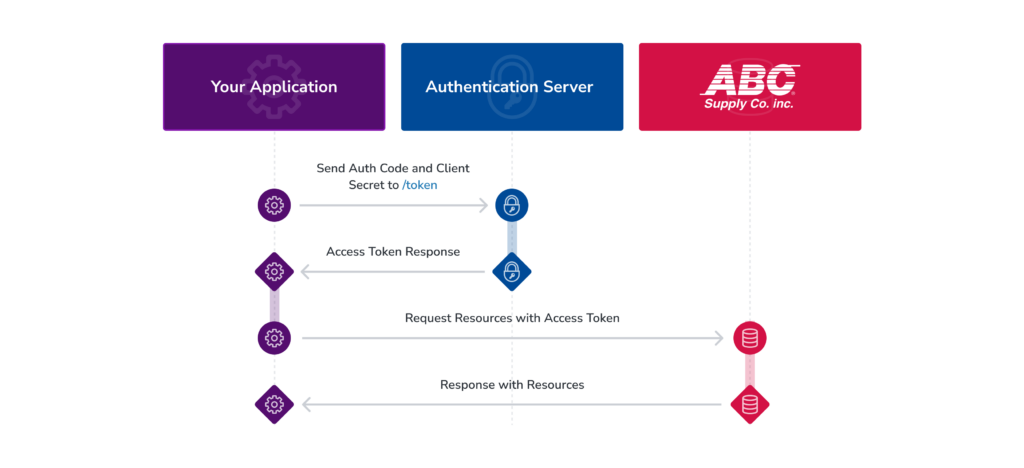
Example Token Request for Production
curl -X POST \
'https://auth.partners.abcsupply.com/oauth2/ausvvp0xuwGKLenYy357/v1/token' \
-H 'Authorization: Basic {Base64(clientId:clientSecret)}' \
-H 'Content-Type: application/x-www-form-urlencoded' \
-d 'grant_type=client_credentials&scope=location.read product.read notification.read notification.write location.read product.read account.read pricing.read allOrder.read order.write notification.read notification.write'
Note: There is no authorization or refresh token call with the Client Credentials flow.
Available Scopes
Scope Name
Description
location.read
Allows access to the Location API
product.read
Allows access to the Products API
account.read
Allows access to the Accounts API
pricing.read
Allows access to the Pricing API
allOrder.read
Allows read-access to Order API for all orders placed with the enrolled ABC account(s)
order.write
Allows write-access to Order API for placing orders
notification.read
Allows read-access for Notification API
notification.write
Allows write-access for Notification API
Token Lifetimes
Access Tokens
Access tokens have a lifetime of 30 minutes. After 30 minutes, the refresh token (valid only for auth code flows) will need to be used to get a new access token and new refresh token.
Refresh Tokens
Refresh tokens have an infinite lifetime as long as they continue to be refreshed. If 30 days pass without the refresh token being used, the user will have to go through the authorization flow again to gain a new access and refresh token.